The C++ Function using STL to Check Duplicate Elements/Character
- Time:2020-09-17 14:37:27
- Class:Weblog
- Read:32
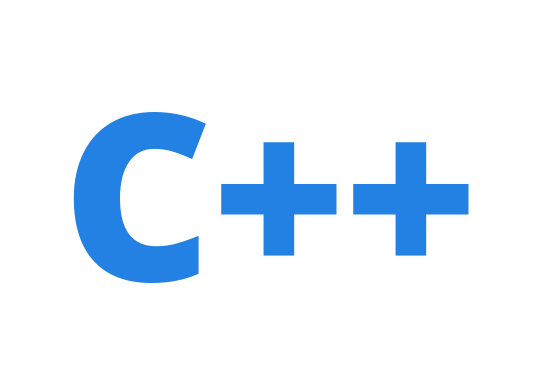
cplusplus
Let’s say we want to implement a C++ function based on STL containers to check if a given string contains duplicate characters, or a given vector/array contains duplicate elements. Luckily we can use the unordered set (or set which maintains order), that we can construct a set based on the vector/array/string, then we just need to compare the sizes of both set and the original container – if they are equal, it means all unique elements or duplicates otherwise.
1 2 3 4 | bool hasDuplicateCharacters(string arr) { unordered_set<char> st(begin(arr), end(arr)); return st.size() != arr.size(); } |
bool hasDuplicateCharacters(string arr) { unordered_set<char> st(begin(arr), end(arr)); return st.size() != arr.size(); }
Example:
1 2 | cout << (hasDuplicateCharacters("abcde") ? "true" : "false"); // false cout << (hasDuplicateCharacters("abcdea") ? "true" : "false"); // true |
cout << (hasDuplicateCharacters("abcde") ? "true" : "false"); // false cout << (hasDuplicateCharacters("abcdea") ? "true" : "false"); // true
We can use the C++ generic that allows us to pass in most data types – using the templates.
1 2 3 4 5 | template <typename T> bool hasDuplicateItems(vector<T> arr) { unordered_set<T> st(begin(arr), end(arr)); return st.size() != arr.size(); } |
template <typename T> bool hasDuplicateItems(vector<T> arr) { unordered_set<T> st(begin(arr), end(arr)); return st.size() != arr.size(); }
Example:
1 2 3 | cout << (hasDuplicateItems<int>({ 1, 2, 3, 4 }) ? "true" : "false"); // true cout << (hasDuplicateItems</int><int>({ 1, 2, 3, 4, 1 }) ? "true" : "false"); // false </int> |
cout << (hasDuplicateItems<int>({ 1, 2, 3, 4 }) ? "true" : "false"); // true cout << (hasDuplicateItems</int><int>({ 1, 2, 3, 4, 1 }) ? "true" : "false"); // false </int>
This is quite similar approach to checking the duplicates in Javascript – which is also based on the Set.
–EOF (The Ultimate Computing & Technology Blog) —
Recommend:Python Coding Reference: index() and find() for string (substrin
Walking Robot Simulation Algorithm with Obstacles Detection
The Selection Sorting Algorithm in VBScript
Find the Queens That Can Attack the King
4 Bloggers Who Were Killed Because Of Their Content
Top Mobile Technology Trends for Bloggers
The Vital Common Sense Marketing Strategy You May Have Forgotten
The 7 Features of a Successful Product Demo Video
Top Facebook Marketing Partner Comments On Facebook’s Newest Vid
Animoto Adds Square Video for Increased Social Media and Mobile
- Comment list
-
- Comment add