Introduction to Microbit and Javascript/Typescript Programming
- Time:2020-09-17 14:26:24
- Class:Weblog
- Read:32
Pre-requisites
The Microbit project can be created in browser: https://makecode.microbit.org
Alternatively, you could download the Microbit App from https://microbit.org/code/
The microbit is currently supported on Windows, Android and iOS.
Introduction to Microbit and Javascript
The microbit is a tiny computer (40mm x 50mm, slightly smaller than a credit card) which has a processor (Nordic Semiconductor nRF51822 16MHz CPU with an ARM Cortex-M0 microcontroller), some RAM (16KB RAM and 256KB flash memory).
Microbit supports 2.4G bluetooth technology so that you can pair it with the computer, ipad or your phones (press A+B buttons, and press and release button to pair).
THe Microbit can be powered via a USB cable. You can also download the program via the USB cable. You can also use a 2xAAA battery (1.5V) to power the Microbit via the battery connector.
The Microbit has a 5×5 (25 pixels) LED screen, and two buttons A and B. It has inbuilt accelerometer (Freescale MMA8652) and a three-axis magnetometer (Freescale MAG3110), which is also a compass.
Javascript/TypeScript Programming
The Microbit can be programmed via Python or Javascript. The program can then be downloaded to Microbit via USB cable or bluetooth. If you are coding in the browser, you can download the program to a HEX file, which then can be sent to Microbit – which looks like a normal USB drive.
The official programming language is Javascript/Typescript, but you can also program in Python: https://python.microbit.org/v/1.1
Javascript (not to confuse with Java – which is a different programming language) is a popular programming language nowadays, especially it dominates the web programming e.g. the web pages that your browse mostly contain the Javascript code. The Javascript can also be used in backend applications e.g. NodeJs.
The Javascript is a subset of Typescript, and in Microbit, since you need to declare the type of the parameters, it is Typescript but we will refer to Javascript in this course to keep it simple.
The Microbit HEX file
Either you program in Javascript or in Python, the code will be compiled to HEX file before sending to Microbit.
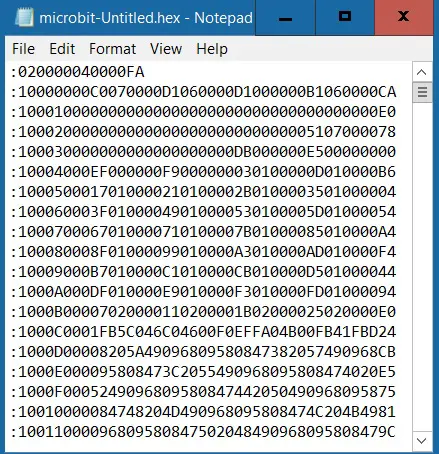
microbit-hex-file
The HEX file is a plain text file but it contains the HEX values which are friendly to the hardware – the microbit.
Creating a New Project in Microbit
Click the “New Project” button to start a new project in Microbit.
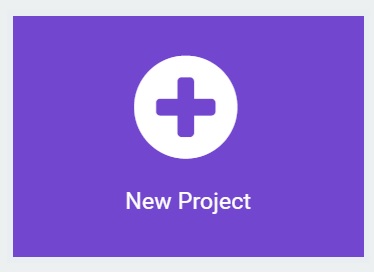
Create a New Project in Microbit App
Then we should see the interface consisting of three parts: a microbit emulator on the left, a toolbar (containing blocks) in the middle, and a visualization area on the right. On the visualization area, there are two modes (Blocks and Javascript) which can be switched from one to another by clicking the button on the top.
We can drag the blocks from the toolbar to the visualization area (the blocks or the Javascript) to program the microbit. When users switch from Blocks to Javascript, the same logics will be translated to Javascript code, and vice versa. However, when Microbit translates to code, the code will lose some good coding styles – removing the optional semi-colons or duplicate code assignments, such as translating “on start, set counter to 1” to the following two lines of code:
1 2 | let counter = 0 counter = 1 |
let counter = 0 counter = 1
instead of the single line assignment:
1 | let counter = 1; |
let counter = 1;
The let keyword is enforced despite that the Javascript can also support traditional var keyword to declare a variable.
To declare a constant, we use the const keyword, for example:
1 | const aNumber = 123; |
const aNumber = 123;
And that means aNumber cannot be altered, it is immutable. If you attempt to change it, the microbit will hint a code error: Cannot assign to ‘aNumber’ because it is a constant or a read-only property.
The if-else, for and while loop in Javascript
We use the if-then or if-then-else to ask the microbit to make judgement based on some condition which returns true or false (boolean values). For example, if today is raining, I am staying home, else I will go out play.
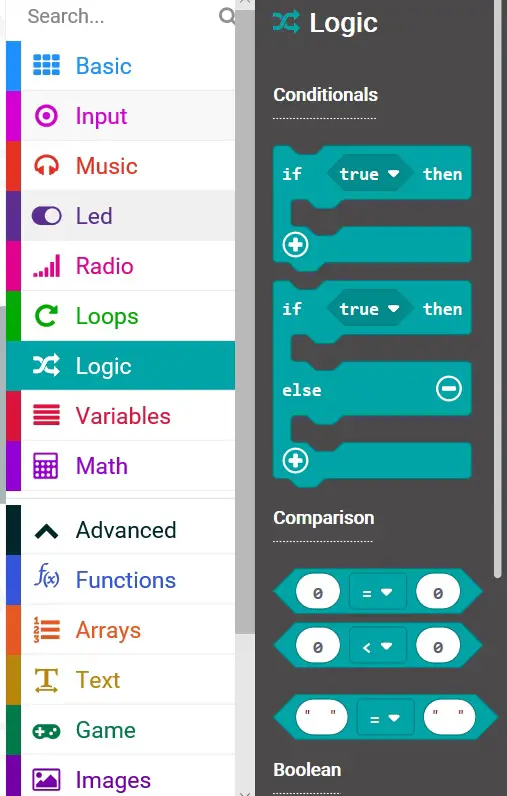
microbit-if-then-else-logics
The for performs a loop and has the following syntax in Javascript:
1 2 3 | for (initialization; condition; post-action) { // loop body } |
for (initialization; condition; post-action) { // loop body }
For example, here is a simple for loop that shows digits from 0 to 10 in Microbit:
1 2 3 | for (let index = 0; index <= 10; index++) { basic.showNumber(index) } |
for (let index = 0; index <= 10; index++) { basic.showNumber(index) }
And this will be visualized as:
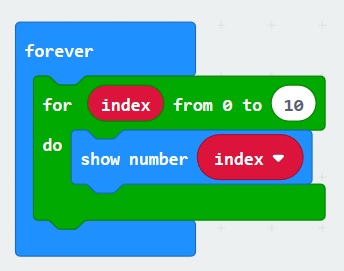
microbit-for-simple-loop
To increment a variable we can use one of the following:
1 2 3 | index ++; index += 1; index = index + 1; |
index ++; index += 1; index = index + 1;
Similarly, to decrement a variable, we can use one of the following:
1 2 3 | index --; index -= 1; index = index - 1; |
index --; index -= 1; index = index - 1;
The while is a keyword for an intuitive loop. While something is true, do something. For example, the following shows numbers smaller than 10.
1 2 3 4 | while (counter < 10) { basic.showNumber(counter) counter += 1 } |
while (counter < 10) { basic.showNumber(counter) counter += 1 }
And it will be visualized in Microbit as:
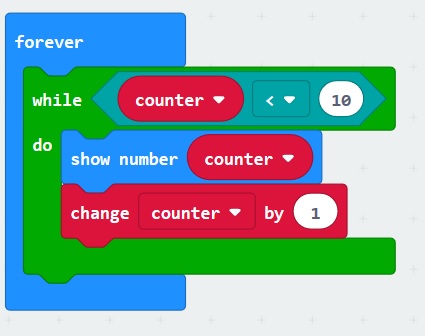
microbit-while-simple-loop
Combining the loop(s), we can let computer (the microbit in this case) perform some calculations as computers are in general faster than humans regarding to doing repetitive tasks e.g. summing up the numbers from 1 to 100, and then show the result in Microbit – the accumulated sum is 5050.
1 2 3 4 5 | let sum = 0; for (let i = 0; i <= 100; i ++) { sum += i; } basic.showNumber(sum); |
let sum = 0; for (let i = 0; i <= 100; i ++) { sum += i; } basic.showNumber(sum);
Finally, we have made a simple counter project that when we press Button A, the counter shown on the Microbit LED screen is increased by one, and when we press the button B, the number is decremented by one, however, when the counter falls below zero, the counter will be updated to 5 instead.
The microbit javascript code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | input.onButtonPressed(Button.A, function () { counter += 1 }) input.onButtonPressed(Button.B, function () { counter += -1 if (counter < 0) { counter = 5 } }) let counter = 0 counter = 1 basic.forever(function () { basic.showNumber(counter) }) |
input.onButtonPressed(Button.A, function () { counter += 1 }) input.onButtonPressed(Button.B, function () { counter += -1 if (counter < 0) { counter = 5 } }) let counter = 0 counter = 1 basic.forever(function () { basic.showNumber(counter) })
And it should visualize as the following:
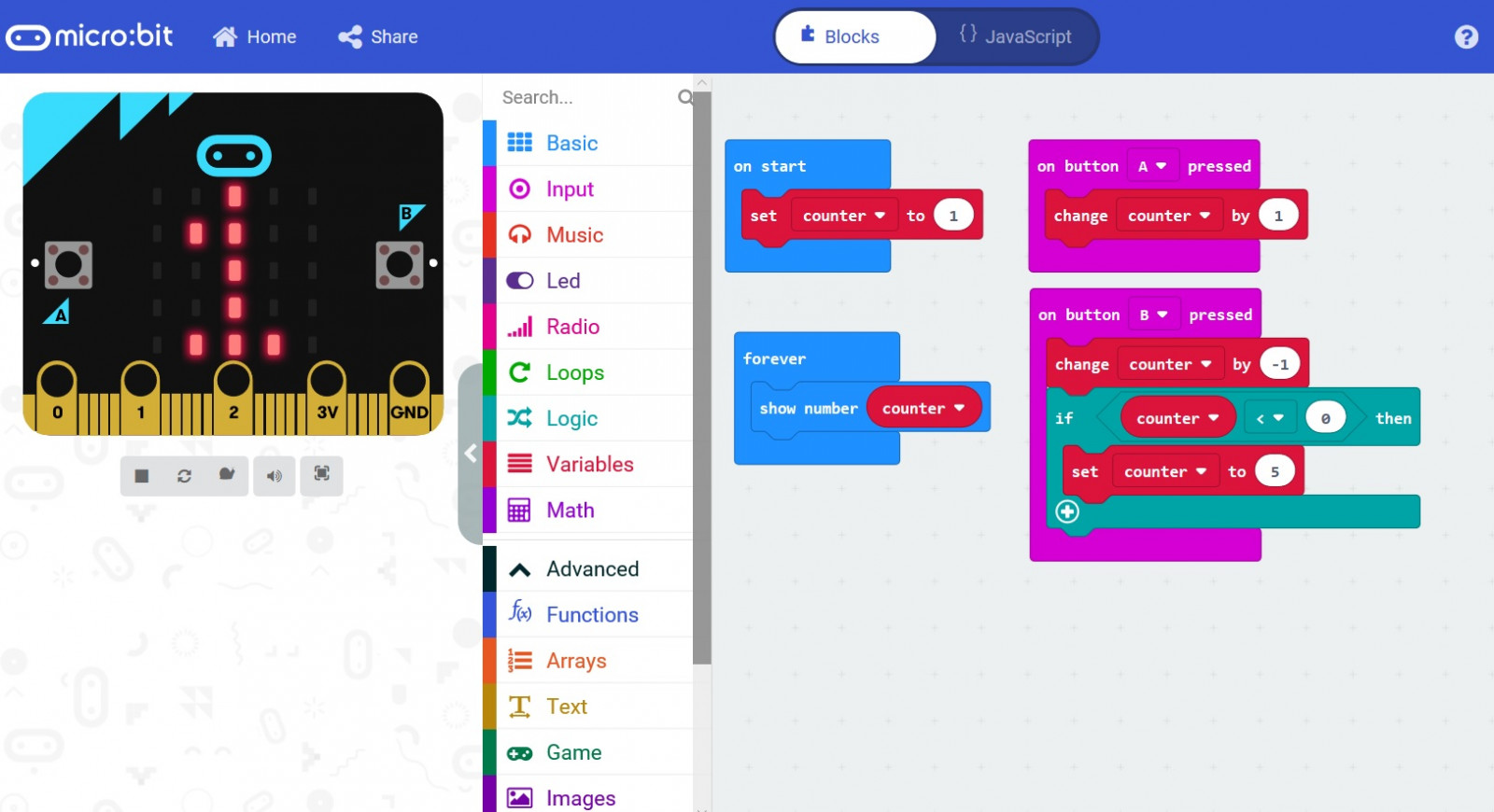
microbit-counter-show-with-buttons
I hope you enjoy programming the Microbit with Javascript, and see you next week: Microbit Programming: Showing a Running Pixel on the LED
–EOF (The Ultimate Computing & Technology Blog) —
Recommend:China mulls law revision to better protect maritime passengers
Shenzhou-18 mission returns samples for extraterrestrial habitat
Indonesian president to visit China
Italian president to visit China
Scenery of Guangwu Mountain scenic area in Sichuan, SW China
Guests attend opening ceremony of World Conference of Classics i
Xi congratulates Maia Sandu on reelection as president of Moldov
China’s central bank confers with foreign financial institutions
Chinese, Peruvian presidents to attend inauguration of Chancay P
China to host world conference on vocational, technical educatio
- Comment list
-
- Comment add