Three ways to Reverse a List/Array/Tuple in Python
- Time:2020-09-16 12:48:17
- Class:Weblog
- Read:14
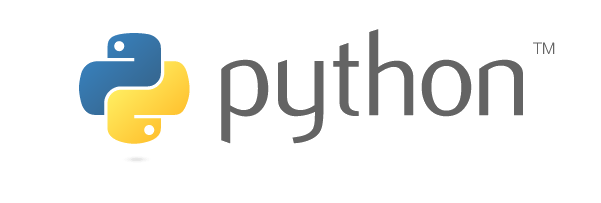
python
Reversing a List/Array is very commonly needed and there are three ways to reverse a list or array in Python.
Using the .reverse() method to reverse a list or array
Note: this method does not work for tuples in Python.
1 2 3 4 5 6 | a (1, 2, 3, 4, 5) >>> a.reverse() Traceback (most recent call last): File "stdin", line 1, in module AttributeError: 'tuple' object has no attribute 'reverse' |
a (1, 2, 3, 4, 5) >>> a.reverse() Traceback (most recent call last): File "stdin", line 1, in module AttributeError: 'tuple' object has no attribute 'reverse'
The .reverse() will modify the given array/list. See the below:
1 2 3 | a = [1, 2, 3, 4, 5] a.reverse() # returns None print(a) # [5, 4, 3, 2, 1] |
a = [1, 2, 3, 4, 5] a.reverse() # returns None print(a) # [5, 4, 3, 2, 1]
As we can see in the above Python code, the .reverse() does not create a copy, rather, it reverses the array/list in-place. The .reverse() method returns None.
Using the slicing to reverse an array, list or tuples in Python
We can use the [::-1] to returns a copy, without modifying the input. It works for list/array and tuples as well.
1 2 3 4 5 6 7 8 9 10 11 | >>> a = (1, 2, 3, 4, 5) (1, 2, 3, 4, 5) >>> a[::-1] (5, 4, 3, 2, 1) >>> a (1, 2, 3, 4, 5) >>> a=[1,2,3,4,5] >>> a[::-1] [5, 4, 3, 2, 1] >>> a [1, 2, 3, 4, 5] |
>>> a = (1, 2, 3, 4, 5) (1, 2, 3, 4, 5) >>> a[::-1] (5, 4, 3, 2, 1) >>> a (1, 2, 3, 4, 5) >>> a=[1,2,3,4,5] >>> a[::-1] [5, 4, 3, 2, 1] >>> a [1, 2, 3, 4, 5]
This is quite cool and the most Pythonic way to reverse a list or tuple.
Python reversed() method to return a reversed iterator
We can use the reversed() function (inbuilt Python) that allows us to return an iterator. As for the list/array:
1 2 3 4 | a = [1, 2, 3, 4, 5] reversed(a) # returns an iterator list(reversed(a)) converts to list [5, 4, 3, 2, 1] tuple(reversed(a)) converts to tuple (5, 4, 3, 2, 1) |
a = [1, 2, 3, 4, 5] reversed(a) # returns an iterator list(reversed(a)) converts to list [5, 4, 3, 2, 1] tuple(reversed(a)) converts to tuple (5, 4, 3, 2, 1)
It works for tuples as well:
1 2 3 4 | a = (1, 2, 3, 4, 5) reversed(a) # returns an iterator list(reversed(a)) converts to list [5, 4, 3, 2, 1] tuple(reversed(a)) converts to tuple (5, 4, 3, 2, 1) |
a = (1, 2, 3, 4, 5) reversed(a) # returns an iterator list(reversed(a)) converts to list [5, 4, 3, 2, 1] tuple(reversed(a)) converts to tuple (5, 4, 3, 2, 1)
Returning a reversed() iterator does not modify the original array, list or tuple as well. When you call list() or tuple(), you can convert the reversed iterator explicitly to a list or tuple.
Oh, it seems years ago I have written on this topics already: Reverse List/Tuple/String in Python
–EOF (The Ultimate Computing & Technology Blog) —
Recommend:4 Ways to Build an Audience for Your New Blog
3 Times Social Media and Cancel Culture Helped in Snuffing Out R
How to Track Your Time as a Freelance Blogger
US Government on Ban TikTok: Should you worry about your blog?
Blogging Mistakes Most Beginner Bloggers Make
Creating a Website for Your Brand: 4 Best Practices You Must Con
What Is Content and Why Does It Matter?
5 Things to Know When Starting a Financial Blog
How to Improve the Marketing for Your Online Business
Building an Online Store – What You Need to Do
- Comment list
-
- Comment add