How to Remove Items/Entries with Specific Values from Map/HashMa
- Time:2020-09-07 12:13:31
- Class:Weblog
- Read:28
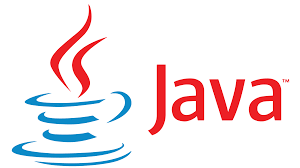
Java
In Java, you can usually remove items or entries with some specific value from Map or HashMap object using traditional method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | public Map<String, String> removeItemByValue(Map<String, String> data, String value) { for (var key: data.keySet()) { if (data.get(key) == null) { data.remove(key); } } return data; } var data = new HashMap<String, String>(); data.put("a", "1"); data.put("b", "2"); data.put("c", "2"); removeItemByValue(data, null); // only prints a = 1 for (var key: data.keySet()) { System.out.println(key + "=" + data.get(key)); } |
public Map<String, String> removeItemByValue(Map<String, String> data, String value) { for (var key: data.keySet()) { if (data.get(key) == null) { data.remove(key); } } return data; } var data = new HashMap<String, String>(); data.put("a", "1"); data.put("b", "2"); data.put("c", "2"); removeItemByValue(data, null); // only prints a = 1 for (var key: data.keySet()) { System.out.println(key + "=" + data.get(key)); }
A Better Map-Item Removal Approach via using values()
The values() method in Map object actually returns a Collection view of the valujes that are contained in the map object. And the remove object return null when the item is not existent anymore. Thefore, we can use the following modern 1-liner in Java to remove the items in a Map that have the specific value.
1 2 3 4 | public Map<String, String> removeItemByValue(Map<String, String> data, String value) { while (data.values().remove(value)) ; return data; } |
public Map<String, String> removeItemByValue(Map<String, String> data, String value) { while (data.values().remove(value)) ; return data; }
To double check and verify that items are actually removed:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | package com.helloacm; import java.util.HashMap; import java.util.Map; public class Main { public static void main(String[] args) { var data = new HashMap<String, String>(); data.put("a", "b"); data.put("e", "2"); data.put("f", "2"); while (data.values().remove("2")); // prints "a" for (Map.Entry<String, String> entry: data.entrySet()) { System.out.println(entry.getKey()); } } } |
package com.helloacm; import java.util.HashMap; import java.util.Map; public class Main { public static void main(String[] args) { var data = new HashMap<String, String>(); data.put("a", "b"); data.put("e", "2"); data.put("f", "2"); while (data.values().remove("2")); // prints "a" for (Map.Entry<String, String> entry: data.entrySet()) { System.out.println(entry.getKey()); } } }
–EOF (The Ultimate Computing & Technology Blog) —
Recommend:What Should You Blog About During the Pandemic and Beyond?
6 Tips For Starting a Business During a Pandemic
Will Your Blog be affected by the Current Facebook Ad Boycott?
Unobvious Life Hacks for Ads Optimization in Google
Essential Traits That Will Help Bloggers Work From Home Successf
Examining the Physical and Mental Health of Writers and Bloggers
How will Blogging Change after the Pandemic?
Digital Relationships: 5 Tips For Building A Better Veterinary B
The 2020 Pandemic Further Boosts Earnings and Influence of Blogg
What to Do When Your Blog Gets Too Big for You Alone
- Comment list
-
- Comment add